Factory Method Pattern
Created: 28 August 2012 Modified:Defines an interface for creating an object, but lets subclasses decide which class to instantiate. Factory method lets a class defer instantiation to subclasses.
Head First Design Patterns
This design pattern will come in handy when we want a character generator that will not only build SuperHeroes but Fantasy Heroes as well. We define an interface and build different hero creators from this interface. These hero creators will then build heroes. All the files can be downloaded.
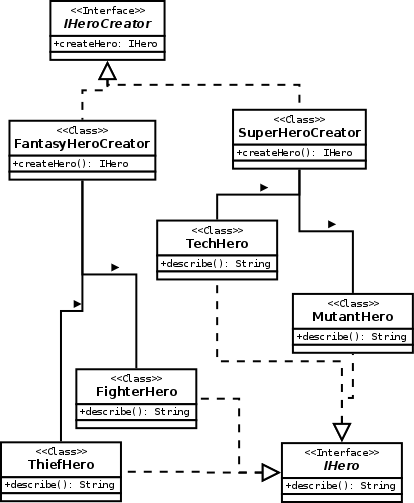
Finally we can build these classes and objects in Ruby as seen in the following code. One difference between the code and the class diagram is that Ruby doesn’t need to define the abstract classes. Nor does it need to define separate creators for the Fantasy and Super Heros such as Java would have to do.
Factory-Method-Pattern.rb
class TechHero
def describe
puts 'I have a suit of wondrous technology!'
end
end
class MutantHero
def describe
puts 'I genetic legacy of wonder!'
end
end
class FighterHero
def describe
puts 'I am an unparalleled warrior!'
end
end
class ThiefHero
def describe
puts 'I an a sneak of no mean skill!'
end
end
class HeroCreator
def createHero(hero_type)
@heroClass = self.class.const_get("#{hero_type}Hero")
@hero = @heroClass.new()
@hero
end
end
creator = HeroCreator.new()
hero = creator.createHero('Tech')
hero.describe
hero = creator.createHero('Mutant')
hero.describe()
hero = creator.createHero('Fighter')
hero.describe()
hero = creator.createHero('Thief')
hero.describe()